-
Notifications
You must be signed in to change notification settings - Fork 89
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
EDU-13381: FastStore SDK - Cart overview #1678
Open
mariana-caetano
wants to merge
3
commits into
main
Choose a base branch
from
EDU-13381
base: main
Could not load branches
Branch not found: {{ refName }}
Loading
Could not load tags
Nothing to show
Loading
Are you sure you want to change the base?
Some commits from the old base branch may be removed from the timeline,
and old review comments may become outdated.
Open
Changes from all commits
Commits
Show all changes
3 commits
Select commit
Hold shift + click to select a range
File filter
Filter by extension
Conversations
Failed to load comments.
Loading
Jump to
Jump to file
Failed to load files.
Loading
Diff view
Diff view
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,92 @@ | ||
--- | ||
title: Cart | ||
createdAt: "2025-01-16T14:47:00.000Z" | ||
--- | ||
|
||
The Cart module offers features to manage the store shopping cart. It handles large orders and complex ecommerce operations, such as marketplace integration, coupons, gift options, and promotions. | ||
|
||
## Cart data storage | ||
|
||
Cart data is saved in the browser's [IndexedDB](https://developer.mozilla.org/en-US/docs/Web/API/IndexedDB_API), ensuring that users' carts remain saved even when they close the browser. The data is structured as follows: | ||
|
||
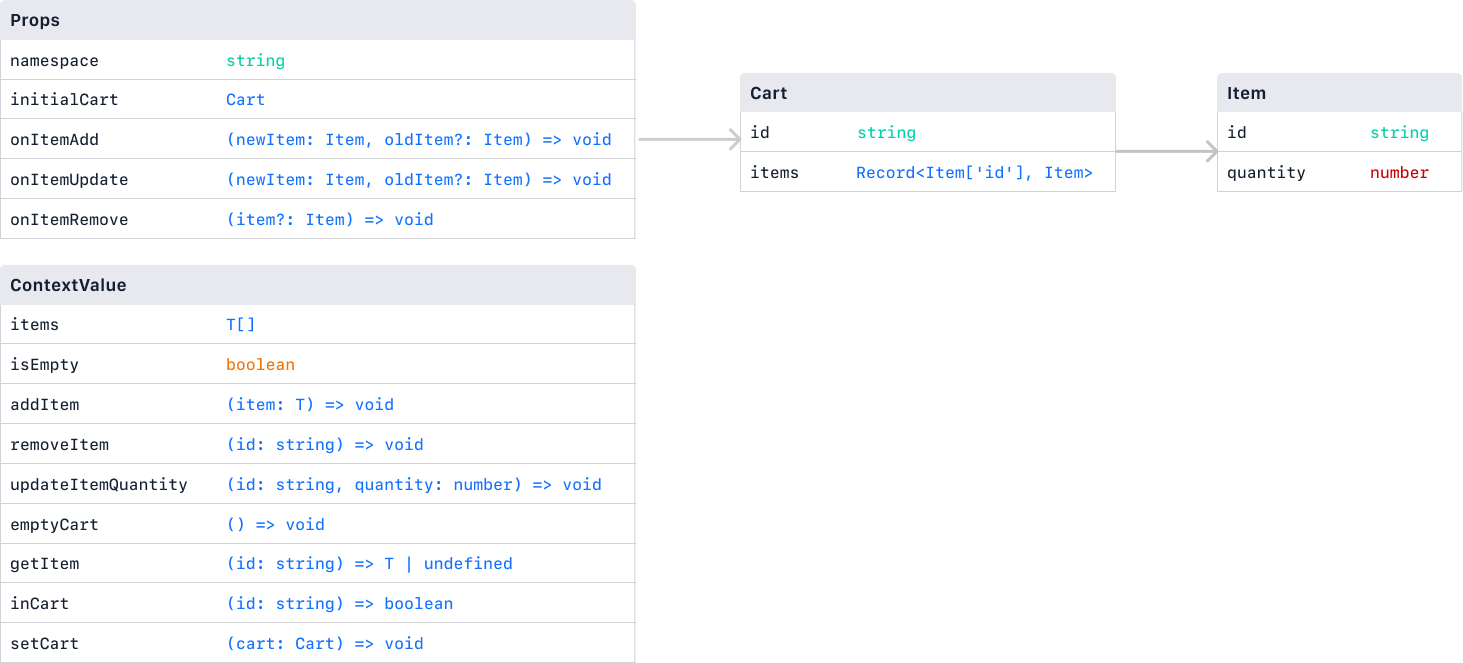 | ||
|
||
## Cart modes | ||
|
||
The Cart module provides two modes: | ||
|
||
- [**Pure**](#pure-mode) (default): Operates on the client side, storing cart data locally and supporting offline use. It lacks server-side validation and may not handle complex cases like unavailable items. Ideal for basic cart functionality. | ||
|
||
- [**Optimistic**](#optimistic-mode): Handles complex ecommerce operations like unavailable items. Validates the cart state on the server using debounced requests. | ||
|
||
## Pure mode | ||
|
||
The Pure mode stores cart data locally in the browser. This allows users to add or remove items and keep their carts even if they close the browser. However, it can't validate items on the server side. | ||
There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. [style-check] reported by reviewdog 🐶 ✍ Check the Education Styleguide for more information. |
||
|
||
The Pure cart also works offline but doesn't automatically correct errors. For example, if a customer adds an out-of-stock item, the cart won't prevent it. The item will appear in the cart even though it's unavailable. | ||
|
||
### Implementing Pure mode | ||
|
||
To implement the Pure cart mode, follow these steps: | ||
|
||
1. Wrap your app with the `CartProvider` component. | ||
2. Access the cart using the `useCart` hook: | ||
|
||
```tsx | ||
import { CartProvider, useCart } from '@faststore/sdk' | ||
|
||
// In the App's root component: | ||
const App = ({ children }) => { | ||
return <CartProvider>{children}</CartProvider> | ||
} | ||
|
||
// In your component: | ||
const MyComponent = () => { | ||
const { items } = useCart() | ||
|
||
return <div>Number of items on cart: {items.length}</div> | ||
} | ||
``` | ||
|
||
## Optimistic mode | ||
|
||
Optimistic mode validates the cart state on the server and handles edge cases like unavailable items. For example, Optimistic mode checks with the store system if a customer tries to add an out-of-stock item. If the product is unavailable, it's removed from the cart, and the customer is notified. | ||
|
||
This feature can be implemented in the `optimistic` mode using the `validateCart` callback function, which allows developers to make requests and cause side effects to the cart. If the function returns `null`, the cart behavior doesn't change. However, if you opt to change the cart state to handle a specific side effect, it must return the new cart state within the callback function. | ||
|
||
### Implementing Optimistic mode | ||
|
||
To implement the Optimistic cart mode, follow these steps: | ||
|
||
1. Use the `CartProvider` component with the `optimistic` mode. | ||
2. Implement the `validateCart` function to handle server-side validation: | ||
|
||
```tsx | ||
import { CartProvider, useCart, Cart } from '@faststore/sdk' | ||
|
||
// In the App's root component: | ||
const validateCart = async (cart: Cart) => { | ||
const response = await fetch(...) | ||
|
||
if (response) { | ||
return response | ||
} | ||
|
||
return null | ||
} | ||
|
||
const App = ({children}) => { | ||
return <CartProvider mode="optimistic" onValidateCart={validateCart}>{children}</CartProvider> | ||
} | ||
|
||
// In your component: | ||
const MyComponent = () => { | ||
const { items, isValidating } = useCart() | ||
|
||
if (isValidating) { | ||
return <div>Cart is validating</div> | ||
} | ||
|
||
return <div>Number items in cart: {items.length}</div> | ||
} | ||
``` |
Oops, something went wrong.
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
[style-check] reported by reviewdog 🐶
❌ Found "close"
Avoid unncessary prepositions.
💡 Suggestion - Replace with "close".