-
Notifications
You must be signed in to change notification settings - Fork 1
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
42 changed files
with
1,480 additions
and
1 deletion.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,33 @@ | ||
class TreeNode { // NOSONAR | ||
constructor(val = 0, left = null, right = null) { | ||
this.val = val | ||
this.left = left | ||
this.right = right | ||
} | ||
}; | ||
|
||
function createTreeNode(treeValues) { | ||
if (treeValues.length === 0) return null | ||
|
||
const root = new TreeNode(treeValues[0]) | ||
const queue = [root] | ||
let i = 1 | ||
|
||
while (i < treeValues.length) { | ||
const curr = queue.shift() | ||
if (treeValues[i] !== null) { | ||
curr.left = new TreeNode(treeValues[i]) | ||
queue.push(curr.left) | ||
} | ||
i++ | ||
if (i < treeValues.length && treeValues[i] !== null) { | ||
curr.right = new TreeNode(treeValues[i]) | ||
queue.push(curr.right) | ||
} | ||
i++ | ||
} | ||
|
||
return root | ||
}; | ||
|
||
export { TreeNode, createTreeNode } |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,27 @@ | ||
56\. Merge Intervals | ||
|
||
Medium | ||
|
||
Given an array of `intervals` where <code>intervals[i] = [start<sub>i</sub>, end<sub>i</sub>]</code>, merge all overlapping intervals, and return _an array of the non-overlapping intervals that cover all the intervals in the input_. | ||
|
||
**Example 1:** | ||
|
||
**Input:** intervals = [[1,3],[2,6],[8,10],[15,18]] | ||
|
||
**Output:** [[1,6],[8,10],[15,18]] | ||
|
||
**Explanation:** Since intervals [1,3] and [2,6] overlap, merge them into [1,6]. | ||
|
||
**Example 2:** | ||
|
||
**Input:** intervals = [[1,4],[4,5]] | ||
|
||
**Output:** [[1,5]] | ||
|
||
**Explanation:** Intervals [1,4] and [4,5] are considered overlapping. | ||
|
||
**Constraints:** | ||
|
||
* <code>1 <= intervals.length <= 10<sup>4</sup></code> | ||
* `intervals[i].length == 2` | ||
* <code>0 <= start<sub>i</sub> <= end<sub>i</sub> <= 10<sup>4</sup></code> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,31 @@ | ||
// #Medium #Top_100_Liked_Questions #Top_Interview_Questions #Array #Sorting | ||
// #Data_Structure_II_Day_2_Array #Level_2_Day_17_Interval #Udemy_2D_Arrays/Matrix | ||
// #Big_O_Time_O(n_log_n)_Space_O(n) #2024_12_10_Time_7_ms_(81.68%)_Space_59.2_MB_(41.78%) | ||
|
||
/** | ||
* @param {number[][]} intervals | ||
* @return {number[][]} | ||
*/ | ||
var merge = function(intervals) { | ||
// Sort intervals based on the starting points | ||
intervals.sort((a, b) => a[0] - b[0]) | ||
|
||
const result = [] | ||
let current = intervals[0] | ||
result.push(current) | ||
|
||
for (const next of intervals) { | ||
if (current[1] >= next[0]) { | ||
// Merge intervals | ||
current[1] = Math.max(current[1], next[1]) | ||
} else { | ||
// Move to the next interval | ||
current = next; | ||
result.push(current) | ||
} | ||
} | ||
|
||
return result; | ||
}; | ||
|
||
export { merge } |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,35 @@ | ||
62\. Unique Paths | ||
|
||
Medium | ||
|
||
There is a robot on an `m x n` grid. The robot is initially located at the **top-left corner** (i.e., `grid[0][0]`). The robot tries to move to the **bottom-right corner** (i.e., `grid[m - 1][n - 1]`). The robot can only move either down or right at any point in time. | ||
|
||
Given the two integers `m` and `n`, return _the number of possible unique paths that the robot can take to reach the bottom-right corner_. | ||
|
||
The test cases are generated so that the answer will be less than or equal to <code>2 * 10<sup>9</sup></code>. | ||
|
||
**Example 1:** | ||
|
||
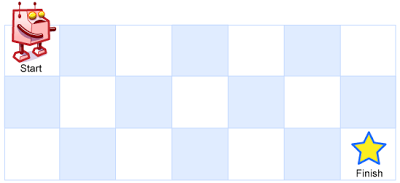 | ||
|
||
**Input:** m = 3, n = 7 | ||
|
||
**Output:** 28 | ||
|
||
**Example 2:** | ||
|
||
**Input:** m = 3, n = 2 | ||
|
||
**Output:** 3 | ||
|
||
**Explanation:** From the top-left corner, there are a total of 3 ways to reach the bottom-right corner: | ||
|
||
1. Right -> Down -> Down | ||
|
||
2. Down -> Down -> Right | ||
|
||
3. Down -> Right -> Down | ||
|
||
**Constraints:** | ||
|
||
* `1 <= m, n <= 100` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,34 @@ | ||
// #Medium #Top_100_Liked_Questions #Top_Interview_Questions #Dynamic_Programming #Math | ||
// #Combinatorics #Algorithm_II_Day_13_Dynamic_Programming #Dynamic_Programming_I_Day_15 | ||
// #Level_1_Day_11_Dynamic_Programming #Big_O_Time_O(m*n)_Space_O(m*n) | ||
// #2024_12_10_Time_0_ms_(100.00%)_Space_49.1_MB_(57.14%) | ||
|
||
/** | ||
* @param {number} m | ||
* @param {number} n | ||
* @return {number} | ||
*/ | ||
var uniquePaths = function(m, n) { | ||
// Initialize a 2D array with all values set to 0 | ||
const dp = Array.from({ length: m }, () => Array(n).fill(0)) | ||
|
||
// Fill the first row and first column with 1 | ||
for (let i = 0; i < m; i++) { | ||
dp[i][0] = 1 | ||
} | ||
for (let j = 0; j < n; j++) { | ||
dp[0][j] = 1 | ||
} | ||
|
||
// Fill the rest of the dp table | ||
for (let i = 1; i < m; i++) { | ||
for (let j = 1; j < n; j++) { | ||
dp[i][j] = dp[i - 1][j] + dp[i][j - 1] | ||
} | ||
} | ||
|
||
// The answer is in the bottom-right corner | ||
return dp[m - 1][n - 1] | ||
}; | ||
|
||
export { uniquePaths } |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,30 @@ | ||
64\. Minimum Path Sum | ||
|
||
Medium | ||
|
||
Given a `m x n` `grid` filled with non-negative numbers, find a path from top left to bottom right, which minimizes the sum of all numbers along its path. | ||
|
||
**Note:** You can only move either down or right at any point in time. | ||
|
||
**Example 1:** | ||
|
||
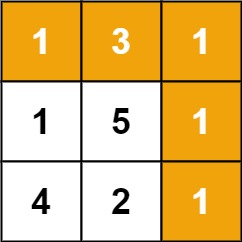 | ||
|
||
**Input:** grid = [[1,3,1],[1,5,1],[4,2,1]] | ||
|
||
**Output:** 7 | ||
|
||
**Explanation:** Because the path 1 → 3 → 1 → 1 → 1 minimizes the sum. | ||
|
||
**Example 2:** | ||
|
||
**Input:** grid = [[1,2,3],[4,5,6]] | ||
|
||
**Output:** 12 | ||
|
||
**Constraints:** | ||
|
||
* `m == grid.length` | ||
* `n == grid[i].length` | ||
* `1 <= m, n <= 200` | ||
* `0 <= grid[i][j] <= 100` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,56 @@ | ||
// #Medium #Top_100_Liked_Questions #Array #Dynamic_Programming #Matrix | ||
// #Dynamic_Programming_I_Day_16 #Udemy_Dynamic_Programming #Big_O_Time_O(m*n)_Space_O(m*n) | ||
// #2024_12_10_Time_3_ms_(83.07%)_Space_51.2_MB_(74.79%) | ||
|
||
/** | ||
* @param {number[][]} grid | ||
* @return {number} | ||
*/ | ||
var minPathSum = function(grid) { | ||
const rows = grid.length | ||
const cols = grid[0].length | ||
|
||
// Handle the special case where grid has only one cell | ||
if (rows === 1 && cols === 1) { | ||
return grid[0][0] | ||
} | ||
|
||
// Create a 2D array for dynamic programming | ||
const dm = Array.from({ length: rows }, () => Array(cols).fill(0)) | ||
|
||
// Initialize the last column | ||
let s = 0 | ||
for (let r = rows - 1; r >= 0; r--) { | ||
dm[r][cols - 1] = grid[r][cols - 1] + s | ||
s += grid[r][cols - 1] | ||
} | ||
|
||
// Initialize the last row | ||
s = 0 | ||
for (let c = cols - 1; c >= 0; c--) { | ||
dm[rows - 1][c] = grid[rows - 1][c] + s | ||
s += grid[rows - 1][c] | ||
} | ||
|
||
// Recursive helper function | ||
const recur = (r, c) => { | ||
if ( | ||
dm[r][c] === 0 && | ||
r !== rows - 1 && | ||
c !== cols - 1 | ||
) { | ||
dm[r][c] = | ||
grid[r][c] + | ||
Math.min( | ||
recur(r + 1, c), | ||
recur(r, c + 1) | ||
) | ||
} | ||
return dm[r][c] | ||
} | ||
|
||
// Start recursion from the top-left corner | ||
return recur(0, 0) | ||
}; | ||
|
||
export { minPathSum } |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,37 @@ | ||
70\. Climbing Stairs | ||
|
||
Easy | ||
|
||
You are climbing a staircase. It takes `n` steps to reach the top. | ||
|
||
Each time you can either climb `1` or `2` steps. In how many distinct ways can you climb to the top? | ||
|
||
**Example 1:** | ||
|
||
**Input:** n = 2 | ||
|
||
**Output:** 2 | ||
|
||
**Explanation:** There are two ways to climb to the top. | ||
|
||
1. 1 step + 1 step | ||
|
||
2. 2 steps | ||
|
||
**Example 2:** | ||
|
||
**Input:** n = 3 | ||
|
||
**Output:** 3 | ||
|
||
**Explanation:** There are three ways to climb to the top. | ||
|
||
1. 1 step + 1 step + 1 step | ||
|
||
2. 1 step + 2 steps | ||
|
||
3. 2 steps + 1 step | ||
|
||
**Constraints:** | ||
|
||
* `1 <= n <= 45` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,31 @@ | ||
// #Easy #Top_100_Liked_Questions #Top_Interview_Questions #Dynamic_Programming #Math #Memoization | ||
// #Algorithm_I_Day_12_Dynamic_Programming #Dynamic_Programming_I_Day_2 | ||
// #Level_1_Day_10_Dynamic_Programming #Udemy_Dynamic_Programming #Big_O_Time_O(n)_Space_O(n) | ||
// #2024_12_10_Time_0_ms_(100.00%)_Space_49_MB_(22.45%) | ||
|
||
/** | ||
* @param {number} n | ||
* @return {number} | ||
*/ | ||
var climbStairs = function(n) { | ||
if (n < 2) { | ||
return n | ||
} | ||
|
||
// Create a cache (DP array) to store results | ||
const cache = new Array(n) | ||
|
||
// Initialize base cases | ||
cache[0] = 1 | ||
cache[1] = 2 | ||
|
||
// Fill the cache using the recurrence relation | ||
for (let i = 2; i < n; i++) { | ||
cache[i] = cache[i - 1] + cache[i - 2] | ||
} | ||
|
||
// Return the result for the nth step | ||
return cache[n - 1] | ||
}; | ||
|
||
export { climbStairs } |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,48 @@ | ||
72\. Edit Distance | ||
|
||
Hard | ||
|
||
Given two strings `word1` and `word2`, return _the minimum number of operations required to convert `word1` to `word2`_. | ||
|
||
You have the following three operations permitted on a word: | ||
|
||
* Insert a character | ||
* Delete a character | ||
* Replace a character | ||
|
||
**Example 1:** | ||
|
||
**Input:** word1 = "horse", word2 = "ros" | ||
|
||
**Output:** 3 | ||
|
||
**Explanation:** | ||
|
||
horse -> rorse (replace 'h' with 'r') | ||
|
||
rorse -> rose (remove 'r') | ||
|
||
rose -> ros (remove 'e') | ||
|
||
**Example 2:** | ||
|
||
**Input:** word1 = "intention", word2 = "execution" | ||
|
||
**Output:** 5 | ||
|
||
**Explanation:** | ||
|
||
intention -> inention (remove 't') | ||
|
||
inention -> enention (replace 'i' with 'e') | ||
|
||
enention -> exention (replace 'n' with 'x') | ||
|
||
exention -> exection (replace 'n' with 'c') | ||
|
||
exection -> execution (insert 'u') | ||
|
||
**Constraints:** | ||
|
||
* `0 <= word1.length, word2.length <= 500` | ||
* `word1` and `word2` consist of lowercase English letters. |
Oops, something went wrong.