[Enhancement] Battery % Display in 1% increments #27
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
So i started out simply trying to shave cycles out of
onBatteryChanged
., mainly by avoiding loops and conversion between number -> string -> number, and ended up completely rewriting it instead to show 101 points of precision in 1% increments, from 0% to 100%. I followed the voltage range "clamps", and wrote a script which calculates the voltage range for each percentage, then generates the code to be placed inonBatteryChanged
.I do not have a cutiepi yet to test this on, so i was hoping the dev team might be willing to test it on a device and ensure it works properly. It should, based on everything i read about QT javascript support
Pros:
Cons:
Wanted to create a jsbench test suite, but the code exceeds the maximum allowed size for a test case.
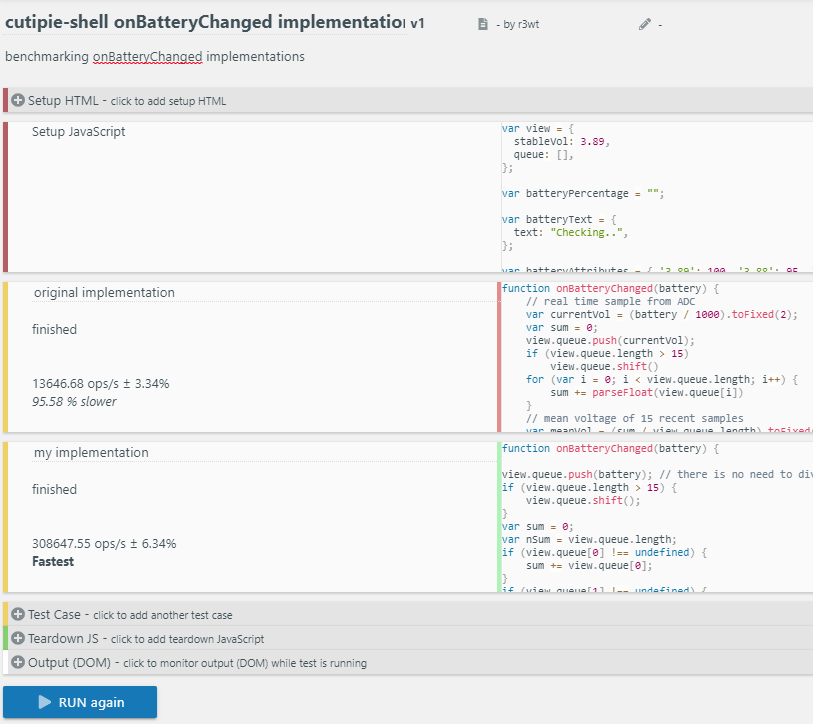
Here's the script i used to generate the code: