-
Notifications
You must be signed in to change notification settings - Fork 1
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
20 changed files
with
751 additions
and
0 deletions.
There are no files selected for viewing
29 changes: 29 additions & 0 deletions
29
src/main/js/g0201_0300/s0230_kth_smallest_element_in_a_bst/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,29 @@ | ||
230\. Kth Smallest Element in a BST | ||
|
||
Medium | ||
|
||
Given the `root` of a binary search tree, and an integer `k`, return _the_ <code>k<sup>th</sup></code> _smallest value (**1-indexed**) of all the values of the nodes in the tree_. | ||
|
||
**Example 1:** | ||
|
||
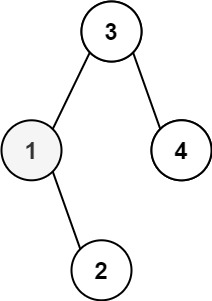 | ||
|
||
**Input:** root = [3,1,4,null,2], k = 1 | ||
|
||
**Output:** 1 | ||
|
||
**Example 2:** | ||
|
||
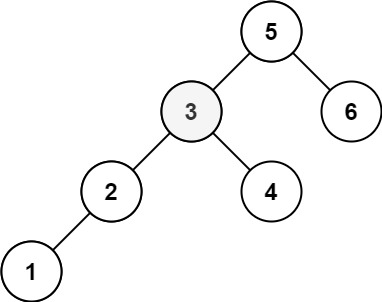 | ||
|
||
**Input:** root = [5,3,6,2,4,null,null,1], k = 3 | ||
|
||
**Output:** 3 | ||
|
||
**Constraints:** | ||
|
||
* The number of nodes in the tree is `n`. | ||
* <code>1 <= k <= n <= 10<sup>4</sup></code> | ||
* <code>0 <= Node.val <= 10<sup>4</sup></code> | ||
|
||
**Follow up:** If the BST is modified often (i.e., we can do insert and delete operations) and you need to find the kth smallest frequently, how would you optimize? |
39 changes: 39 additions & 0 deletions
39
src/main/js/g0201_0300/s0230_kth_smallest_element_in_a_bst/solution.js
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,39 @@ | ||
// #Medium #Top_100_Liked_Questions #Depth_First_Search #Tree #Binary_Tree #Binary_Search_Tree | ||
// #Data_Structure_II_Day_17_Tree #Level_2_Day_9_Binary_Search_Tree #Big_O_Time_O(n)_Space_O(n) | ||
// #2024_12_21_Time_0_ms_(100.00%)_Space_55.4_MB_(48.16%) | ||
|
||
/** | ||
* Definition for a binary tree node. | ||
* function TreeNode(val, left, right) { | ||
* this.val = (val===undefined ? 0 : val) | ||
* this.left = (left===undefined ? null : left) | ||
* this.right = (right===undefined ? null : right) | ||
* } | ||
*/ | ||
/** | ||
* @param {TreeNode} root | ||
* @param {number} k | ||
* @return {number} | ||
*/ | ||
var kthSmallest = function(root, k) { | ||
let count = 0 | ||
let result = null | ||
|
||
const calculate = (node) => { | ||
if (!node) return | ||
if (node.left) calculate(node.left) | ||
|
||
count++ | ||
if (count === k) { | ||
result = node.val | ||
return | ||
} | ||
|
||
if (node.right) calculate(node.right) | ||
} | ||
|
||
calculate(root) | ||
return result | ||
}; | ||
|
||
export { kthSmallest } |
28 changes: 28 additions & 0 deletions
28
src/main/js/g0201_0300/s0234_palindrome_linked_list/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,28 @@ | ||
234\. Palindrome Linked List | ||
|
||
Easy | ||
|
||
Given the `head` of a singly linked list, return `true` _if it is a palindrome or_ `false` _otherwise_. | ||
|
||
**Example 1:** | ||
|
||
 | ||
|
||
**Input:** head = [1,2,2,1] | ||
|
||
**Output:** true | ||
|
||
**Example 2:** | ||
|
||
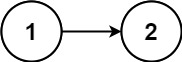 | ||
|
||
**Input:** head = [1,2] | ||
|
||
**Output:** false | ||
|
||
**Constraints:** | ||
|
||
* The number of nodes in the list is in the range <code>[1, 10<sup>5</sup>]</code>. | ||
* `0 <= Node.val <= 9` | ||
|
||
**Follow up:** Could you do it in `O(n)` time and `O(1)` space? |
54 changes: 54 additions & 0 deletions
54
src/main/js/g0201_0300/s0234_palindrome_linked_list/solution.js
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,54 @@ | ||
// #Easy #Top_100_Liked_Questions #Two_Pointers #Stack #Linked_List #Recursion | ||
// #Level_2_Day_3_Linked_List #Udemy_Linked_List #Big_O_Time_O(n)_Space_O(1) | ||
// #2024_12_21_Time_3_ms_(93.71%)_Space_69.8_MB_(76.50%) | ||
|
||
/** | ||
* Definition for singly-linked list. | ||
* function ListNode(val, next) { | ||
* this.val = (val===undefined ? 0 : val) | ||
* this.next = (next===undefined ? null : next) | ||
* } | ||
*/ | ||
/** | ||
* @param {ListNode} head | ||
* @return {boolean} | ||
*/ | ||
var isPalindrome = function(head) { | ||
let len = 0 | ||
let right = head | ||
|
||
// Calculate the length of the linked list | ||
while (right !== null) { | ||
right = right.next | ||
len++ | ||
} | ||
|
||
// Move to the start of the second half of the list | ||
len = Math.floor(len / 2) | ||
right = head | ||
for (let i = 0; i < len; i++) { | ||
right = right.next | ||
} | ||
|
||
// Reverse the right half of the list | ||
let prev = null | ||
while (right !== null) { | ||
let next = right.next | ||
right.next = prev | ||
prev = right | ||
right = next | ||
} | ||
|
||
// Compare the left half and the reversed right half | ||
for (let i = 0; i < len; i++) { | ||
if (prev !== null && head.val === prev.val) { | ||
head = head.next | ||
prev = prev.next | ||
} else { | ||
return false | ||
} | ||
} | ||
return true | ||
}; | ||
|
||
export { isPalindrome } |
41 changes: 41 additions & 0 deletions
41
src/main/js/g0201_0300/s0236_lowest_common_ancestor_of_a_binary_tree/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,41 @@ | ||
236\. Lowest Common Ancestor of a Binary Tree | ||
|
||
Medium | ||
|
||
Given a binary tree, find the lowest common ancestor (LCA) of two given nodes in the tree. | ||
|
||
According to the [definition of LCA on Wikipedia](https://en.wikipedia.org/wiki/Lowest_common_ancestor): “The lowest common ancestor is defined between two nodes `p` and `q` as the lowest node in `T` that has both `p` and `q` as descendants (where we allow **a node to be a descendant of itself**).” | ||
|
||
**Example 1:** | ||
|
||
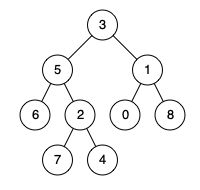 | ||
|
||
**Input:** root = [3,5,1,6,2,0,8,null,null,7,4], p = 5, q = 1 | ||
|
||
**Output:** 3 | ||
|
||
**Explanation:** The LCA of nodes 5 and 1 is 3. | ||
|
||
**Example 2:** | ||
|
||
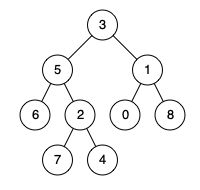 | ||
|
||
**Input:** root = [3,5,1,6,2,0,8,null,null,7,4], p = 5, q = 4 | ||
|
||
**Output:** 5 | ||
|
||
**Explanation:** The LCA of nodes 5 and 4 is 5, since a node can be a descendant of itself according to the LCA definition. | ||
|
||
**Example 3:** | ||
|
||
**Input:** root = [1,2], p = 1, q = 2 | ||
|
||
**Output:** 1 | ||
|
||
**Constraints:** | ||
|
||
* The number of nodes in the tree is in the range <code>[2, 10<sup>5</sup>]</code>. | ||
* <code>-10<sup>9</sup> <= Node.val <= 10<sup>9</sup></code> | ||
* All `Node.val` are **unique**. | ||
* `p != q` | ||
* `p` and `q` will exist in the tree. |
33 changes: 33 additions & 0 deletions
33
src/main/js/g0201_0300/s0236_lowest_common_ancestor_of_a_binary_tree/solution.js
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,33 @@ | ||
// #Medium #Top_100_Liked_Questions #Depth_First_Search #Tree #Binary_Tree | ||
// #Data_Structure_II_Day_18_Tree #Udemy_Tree_Stack_Queue #Big_O_Time_O(n)_Space_O(n) | ||
// #2024_12_21_Time_53_ms_(98.59%)_Space_58.7_MB_(88.33%) | ||
|
||
/** | ||
* Definition for a binary tree node. | ||
* function TreeNode(val) { | ||
* this.val = val; | ||
* this.left = this.right = null; | ||
* } | ||
*/ | ||
/** | ||
* @param {TreeNode} root | ||
* @param {TreeNode} p | ||
* @param {TreeNode} q | ||
* @return {TreeNode} | ||
*/ | ||
var lowestCommonAncestor = function(root, p, q) { | ||
if(!root) { | ||
return null | ||
} | ||
if(p.val === root.val || q.val === root.val) { | ||
return root | ||
} | ||
const leftNode = lowestCommonAncestor(root.left, p, q) | ||
const rightNode = lowestCommonAncestor(root.right, p, q) | ||
if(leftNode && rightNode) { | ||
return root | ||
} | ||
return leftNode || rightNode | ||
}; | ||
|
||
export { lowestCommonAncestor } |
29 changes: 29 additions & 0 deletions
29
src/main/js/g0201_0300/s0238_product_of_array_except_self/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,29 @@ | ||
238\. Product of Array Except Self | ||
|
||
Medium | ||
|
||
Given an integer array `nums`, return _an array_ `answer` _such that_ `answer[i]` _is equal to the product of all the elements of_ `nums` _except_ `nums[i]`. | ||
|
||
The product of any prefix or suffix of `nums` is **guaranteed** to fit in a **32-bit** integer. | ||
|
||
You must write an algorithm that runs in `O(n)` time and without using the division operation. | ||
|
||
**Example 1:** | ||
|
||
**Input:** nums = [1,2,3,4] | ||
|
||
**Output:** [24,12,8,6] | ||
|
||
**Example 2:** | ||
|
||
**Input:** nums = [-1,1,0,-3,3] | ||
|
||
**Output:** [0,0,9,0,0] | ||
|
||
**Constraints:** | ||
|
||
* <code>2 <= nums.length <= 10<sup>5</sup></code> | ||
* `-30 <= nums[i] <= 30` | ||
* The product of any prefix or suffix of `nums` is **guaranteed** to fit in a **32-bit** integer. | ||
|
||
**Follow up:** Can you solve the problem in `O(1) `extra space complexity? (The output array **does not** count as extra space for space complexity analysis.) |
28 changes: 28 additions & 0 deletions
28
src/main/js/g0201_0300/s0238_product_of_array_except_self/solution.js
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,28 @@ | ||
// #Medium #Top_100_Liked_Questions #Array #Prefix_Sum #Data_Structure_II_Day_5_Array #Udemy_Arrays | ||
// #Big_O_Time_O(n^2)_Space_O(n) #2024_12_21_Time_3_ms_(93.60%)_Space_64.9_MB_(45.67%) | ||
|
||
/** | ||
* @param {number[]} nums | ||
* @return {number[]} | ||
*/ | ||
var productExceptSelf = function(nums) { | ||
const res = new Array(nums.length).fill(1) | ||
|
||
// Compute prefix product | ||
let prefixProduct = 1 | ||
for (let i = 0; i < nums.length; i++) { | ||
res[i] = prefixProduct | ||
prefixProduct *= nums[i] | ||
} | ||
|
||
// Compute suffix product and multiply with prefix product | ||
let suffixProduct = 1 | ||
for (let i = nums.length - 1; i >= 0; i--) { | ||
res[i] *= suffixProduct | ||
suffixProduct *= nums[i] | ||
} | ||
|
||
return res | ||
}; | ||
|
||
export { productExceptSelf } |
43 changes: 43 additions & 0 deletions
43
src/main/js/g0201_0300/s0239_sliding_window_maximum/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,43 @@ | ||
239\. Sliding Window Maximum | ||
|
||
Hard | ||
|
||
You are given an array of integers `nums`, there is a sliding window of size `k` which is moving from the very left of the array to the very right. You can only see the `k` numbers in the window. Each time the sliding window moves right by one position. | ||
|
||
Return _the max sliding window_. | ||
|
||
**Example 1:** | ||
|
||
**Input:** nums = [1,3,-1,-3,5,3,6,7], k = 3 | ||
|
||
**Output:** [3,3,5,5,6,7] | ||
|
||
**Explanation:** | ||
|
||
Window position Max | ||
|
||
--------------- ----- | ||
|
||
[1 3 -1] -3 5 3 6 7 **3** | ||
|
||
1 [3 -1 -3] 5 3 6 7 **3** | ||
|
||
1 3 [-1 -3 5] 3 6 7 **5** | ||
|
||
1 3 -1 [-3 5 3] 6 7 **5** | ||
|
||
1 3 -1 -3 [5 3 6] 7 **6** | ||
|
||
1 3 -1 -3 5 [3 6 7] **7** | ||
|
||
**Example 2:** | ||
|
||
**Input:** nums = [1], k = 1 | ||
|
||
**Output:** [1] | ||
|
||
**Constraints:** | ||
|
||
* <code>1 <= nums.length <= 10<sup>5</sup></code> | ||
* <code>-10<sup>4</sup> <= nums[i] <= 10<sup>4</sup></code> | ||
* `1 <= k <= nums.length` |
59 changes: 59 additions & 0 deletions
59
src/main/js/g0201_0300/s0239_sliding_window_maximum/solution.js
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,59 @@ | ||
// #Hard #Top_100_Liked_Questions #Array #Heap_Priority_Queue #Sliding_Window #Queue | ||
// #Monotonic_Queue #Udemy_Arrays #Big_O_Time_O(n*k)_Space_O(n+k) | ||
// #2024_12_21_Time_28_ms_(98.27%)_Space_83.4_MB_(17.86%) | ||
|
||
/** | ||
* @param {number[]} nums | ||
* @param {number} k | ||
* @return {number[]} | ||
*/ | ||
var maxSlidingWindow = function (nums, k) { | ||
// initialize an empty deqeue | ||
let dq = [] | ||
// initailize two pointers with 0 | ||
let i = 0, j = 0 | ||
|
||
// intiailize an empty result variable | ||
let res = [] | ||
|
||
// loop till j reaches to the end of loop | ||
while (j < nums.length) { | ||
|
||
// if dq is empty, push j | ||
if (dq.length === 0) { | ||
dq.push(j) | ||
} else { | ||
// if dq is non empty & back element in deqeue is less or equals to nums[j], | ||
// pop back element from deqeue | ||
while (dq.length !== 0 && nums[dq[dq.length - 1]] <= nums[j]) { | ||
dq.pop() | ||
} | ||
|
||
// push j in deqeue | ||
dq.push(j) | ||
} | ||
|
||
// if current window size is equals to k, | ||
// push front deqeue element in res | ||
if ((j - i + 1) === k) { | ||
res.push(nums[dq[0]]) | ||
|
||
// if front deqeue element is equals to i, | ||
// remove front deqeue element | ||
if (dq[0] === i) { | ||
dq.shift() | ||
} | ||
|
||
// increment i by 1 | ||
i++ | ||
} | ||
|
||
// increment j by 1 | ||
j++ | ||
} | ||
|
||
// return result | ||
return res | ||
}; | ||
|
||
export { maxSlidingWindow } |
Oops, something went wrong.